FastAPI for Scalable Microservices: Architecture, Optimisation, and Best Practices
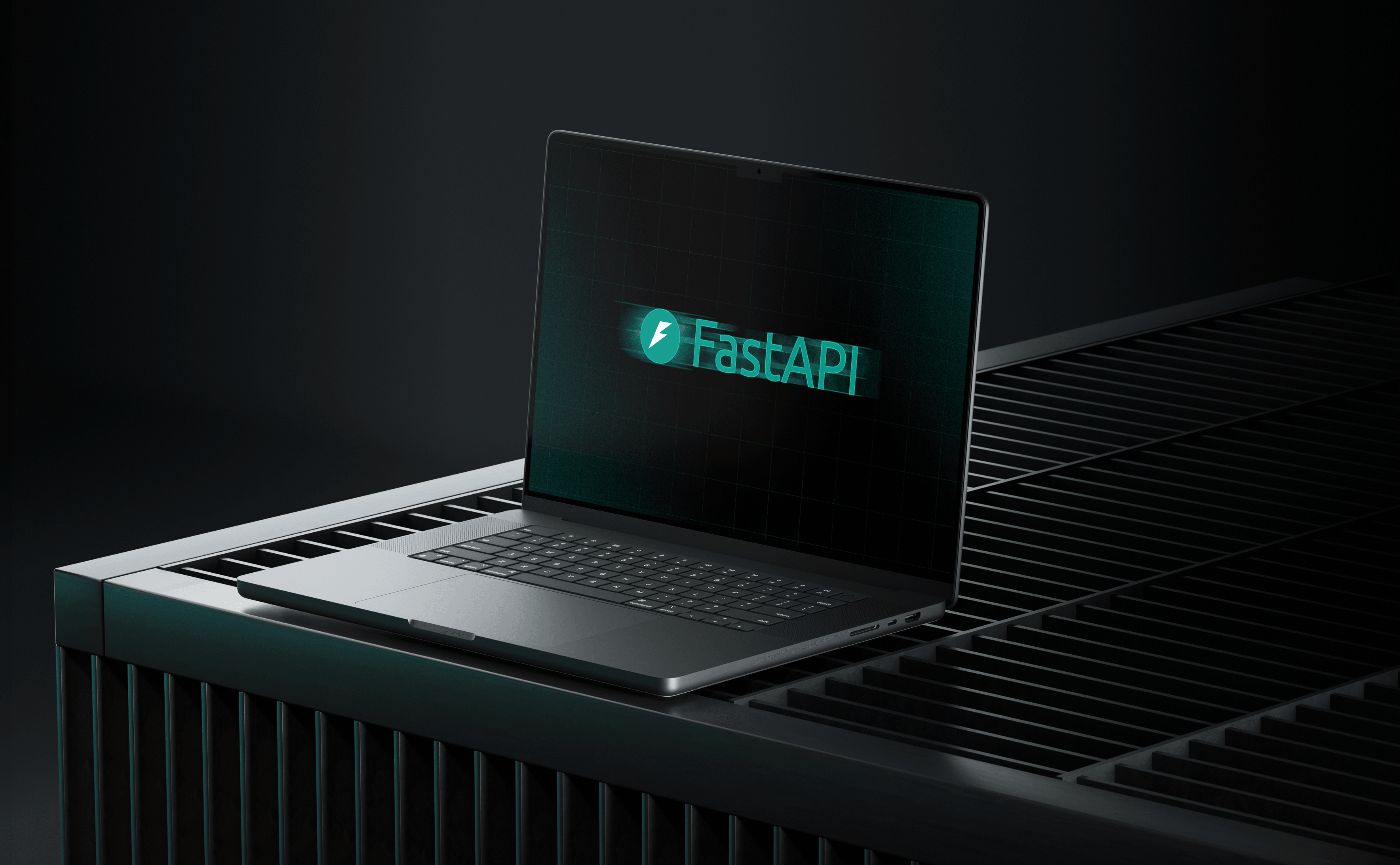
- Why Go for FastAPI for Microservices?
- What are the Best Practices in Microservice Architecture with FastAPI?
- Efficient API Design Using FastAPI
- Authentication & Authorisation in Microservices
- Performance Optimisation Techniques
- Testing & Deployment Strategies
- Understanding CI/CD Workflow
- Microservices with FastAPI: Use Cases
- Conclusion
- Why Go for FastAPI for Microservices?
- What are the Best Practices in Microservice Architecture with FastAPI?
- Efficient API Design Using FastAPI
- Authentication & Authorisation in Microservices
- Performance Optimisation Techniques
- Testing & Deployment Strategies
- Understanding CI/CD Workflow
- Microservices with FastAPI: Use Cases
- Conclusion
Microservices architecture has been prevalent due to its capability to create maintainable, scalable and highly flexible applications. The modularity and independence of Microservices have been favourable in building high-performance systems. FastAPI is well-known for speed and asynchronous tasks and is best suited for microservices, unifying operational and technical efficiencies required to meet advanced requirements. This blog dives into why FastAPI excels in the domain, with the best practices and performance optimisations necessary to building scalable and resilient microservices.
Why Go for FastAPI for Microservices?
FastAPI offers a multitude of benefits to build microservices which range from high performance, usability, and robust support toward asynchronous programming. It also includes built features that favour data validation, interactive documentation, and dependency injection. This makes it a versatile tool to build and maintain microservices. Let’s take a glance at major features that support the use of FastAPI for microservices.
- High Performance: With asynchronous programming, it manages multiple requests concurrently. This improves resource utilisation and minimises response times.
- Intuitive Design: Using Pydantic for data validation, FastAPI makes handling complex data models straightforward while reducing developer errors to maintain reliable data handling.
- Automatic Documentation: By auto-generating interactive API documentation using the integrated Swagger and ReDoc support, FastAPI makes working with microservices efficient.
- Type Hints: Type Hints offer code clarity and minimise runtime errors. This enhances IDE support and makes codebase maintenance easier.
What are the Best Practices in Microservice Architecture with FastAPI?
An optimal FastAPI microservice demands strict adherence to architectural principles. Read to learn and understand the best practices to consider in this context:
Layered Architecture: Organise the code into distinct layers: API, data access, and business logic. It creates modularity and makes every layer evolve independently, for better scalability and maintainability.
Microservice Independence: Every microservice needs its codebase and database, building loosely coupled services that can be updated without relying on the other components.
Domain-Driven Design: By leveraging Domain-Driven Design principles, you can be sure that every microservice closely aligns with specific business domains, minimising unnecessary inter-service communication and encouraging cohesion within every service.
The architecture elevates scalability and maintainability, enabling microservices to evolve independently within the ecosystem.
Efficient API Design Using FastAPI
FastAPI has a design philosophy that promotes developers’ productivity and efficiency. Let’s look at the strategies that can maximise API design:
- Pydantic Models: Pydantic models help with data validation and ensure precise control over data, thereby improving API reliability and security.
- OpenAPI Documentation: The automatic OpenAPI generation simplifies API consumption. Customising the documents enhances readability and ensures alignment with business demands.
- Versioning: Execute API versioning to facilitate backward compatibility, enabling continuous upgrades without changing the existing functionality.
Authentication & Authorisation in Microservices
Since vulnerability in a single point of service can possibly compromise the whole system, security remains primary to the microservice design. FastAPI provides robust tools that support authentication and access control:
- OAuth2 and JWT: Using OAuth2 and JWT support, FastAPI enables secure and scalable token-based authentication.
- Permission Scopes: Define granular permissions to control access depending on user roles, avoiding unauthorised data exposure.
- CORS (Cross-Origin Resource Sharing) Management: Configurable CORS settings offer secure cross-domain interactions, which is crucial for a distributed architecture.
Performance Optimisation Techniques
Performance optimisation is the key factor in high-traffic applications. FastAPI’s asynchronous design offers a quick advantage, but let’s see some additional strategies to manage substantial traffic with minimal latency.
- Async Requests: Asynchronous I/O lets the server handle multiple requests without blocking, enhancing response times and throughput.
- Caching: Caching commonly requested data minimises database load, enabling faster data retrieval.
- Bulk Data Processing: Batch processing for data-intensive endpoints reduces network trips, making large data transactions quicker.
- Database Optimisation: With indexing and connection pooling, you can reduce database access times, and maintain a steady flow of requests.
Testing & Deployment Strategies
You can maintain the quality of production with a focus on testing and deploying microservices. Let’s take a look at the key strategies to be adopted to follow an efficient testing and deployment process.
Automated Testing: FastAPI’s test client and pytest provide tools to implement automated testing and integration testing, with service reliability.
- Containerisation Using Docker: Docker containers follow consistency across environments, which makes deployments quicker and minimises unexpected issues.
- CI/CD Pipelines: With automated CI/CD pipelines, you can minimise manual intervention, thereby enhancing the speed and reliability of deployments.
- Kubernetes Orchestration: Kubernetes offers automatic scaling and recovery in larger architectures, maintaining service uptime in variable loads.
Understanding CI/CD Workflow
An overview of how the CI/CD pipeline workflow functions:
1. Push Code to Repository: Developers push their code to the version control repository. It triggers the pipeline and kicks off a series of automated workflows right away, the code changes are created, to make integration and delivery processes seamless.
2. Run Automated Tests: The system automatically implements tests to verify that the recent code doesn’t bring errors or break the application. It simply validates the code's integrity.
3. Build a Docker Image: A docker image that packages the application and dependencies into a container gets created. This portable image ensures consistent deployment across environments.
4. Deploy to Kubernetes: The containerised app is deployed to a Kubernetes cluster, thus managing the app’s scaling, service availability, and load balancing.
Microservices with FastAPI: Use Cases
Let’s look at some use cases that demonstrate how quickly FastAPI’s type-safe, asynchronous and performance-driven design lets microservices deliver speed and reliability for a range of applications with huge impact.
1. E-commerce Platforms
- Inventory Management: Individual microservices to implement inventory management, stock level tracking, and reorder management to ensure accuracy and avoid out-of-stock circumstances.
- Order Processing: Isolated microservices for every stage of order processing (say, order validation, shipment tracking, payment processing) to serve modular scalability.
- Recommendation Engines: FastAPI’s async features can manage high-volume recommendation requests, delivering real-time personalised product suggestions.
2. Real-Time Analytics and Monitoring
- Data Ingestion Services: FastAPI microservices ingest data streams in real-time, which is ideal for applications that demand quick insights like IoT data, or user behaviour monitoring.
- Metrics Dashboards: Microservices aggregates and serves analytics data for dashboards, enabling fast, asynchronous updates on KPIs and metrics.
3. Social Media and Messaging Applications
- User Profiles & Authentication: FastAPI’s native support for OAuth2 and JWT authentication makes it ideal to securely manage user sessions and permissions.
- Messaging Services: With FastAPI's potential to handle concurrent requests with low latency, you can make message delivery, chat, etc., efficient.
- Notification Systems: The async support FastAPI simplifies sharing real-time push notifications or alerts to users while ensuring quick delivery and reduced delays.
4. Financial and Banking Services
- Transaction Processing: FastAPI microservices manage transaction workflows like calculations, validations, and notifications, ensuring secure and low-latency processing to serve banking applications.
- Risk and Fraud Detection: Asynchronous processing lets FastAPI manage huge volumes of transaction data, flagging anomalies in real time and integrating with ML (machine learning) models.
- Account Handling: FastAPI helps streamline your account management to enable efficient management of user-centric processes such as authentication, registration, profile and account updates, etc.
5. Healthcare and Telemedicine
- Real-time Consultation Platforms: FastAPI’s async requests help with real-time doctor and patient communication and health tracking, ideal in telemedicine applications.
- Prescription and Order Processing: Scalable microservice manages prescriptions and medication orders with credible status tracking and audit trails.
- Patient Data Management: HIPAA-compliant microservices manage patient records securely. It makes access to patient-specific data, appointments, etc., efficient.
6. Streaming and Content Delivery Services
- Video Content Catalogs: Microservices are useful to manage video metadata, categorisation and recommendations for users, with async processing and efficient caching to support huge traffic.
- Subscription and Billing Management: Microservices can manage subscription renewals, payment processing, and billing calculations, whereas FastAPI’s built-in data validation helps with secure transactions.
- User Profiles & Recommendations: FastAPI-powered recommendation engines can process preferences and offer real-time personalised content recommendations.
7. Travel and Transportation Platforms
- Booking Systems: Microservices handle cancellations, reservations, and modifications independently using FastAPI’s async support that ensures responsiveness.
- Real-time Vehicle Tracking: FastAPI microservices manage GPS updates in real-time for logistics or ride-hailing by delivering accurate location data to the users.
- Pricing and Availability Engines: Dynamic pricing and availability can be managed with scalable microservices, which adjust seat availability and fares based on real-time updates and demand.
8. Machine Learning Model Serving
- Model Inference Services: The async capabilities of FastAPI make it a reliable choice to serve machine learning models as microservices, thereby helping with low latency inference considering data-driven applications.
- Data Preprocessing Pipelines: Microservices can handle data validation, feature extraction and transformation, helping to feed pre-processed data models in real-time.
- A/B Testing & Experimentation: FastAPI microservices support experimentation frameworks, helping to test algorithms or models to perform with minimum latency.
9. Event-Driven Systems and Notifications
- Email & SMS Notifications: FastAPI can manage event-triggered notifications in real-time, and share emails, SMS, or push notifications to users as per their specific needs or thresholds.
- Webhooks and Callbacks: Microservices can listen for webhook events from external services (like third-party data updates, or payment confirmations), and go for asynchronous processing for prompt updates.
- Event Logging & Monitoring: Asynchronous microservices can log and track user actions or system events in real-time, and they offer data for analytics or incident responses.
Conclusion
FastAPI takes an advanced approach to microservices, offering flexibility, speed and streamlined development. With asynchronous features, security options, and built-in documentation, FastAPI is a suitable choice for building scalable and highly performing microservices that fit the business’s technical requirements.
FastAPI microservices can thrive in any production environment, provided these adhere to the best practices encompassing architecture, security, API design and CI/CD. With FastAPI in microservices, developers can adopt a framework that emphasises simplicity combined with advanced functionality. This approach lets microservices transform into integral components with a focus on a resilient app ecosystem. As a seasoned Python development company, we specialize in building scalable and efficient microservices with FastAPI. Reach out to us today!
Curious about building scalable microservices?
Let's talkLoading...
Discover Digital Transformation
Please feel free to share your thoughts and we can discuss it over a cup of tea.