Developing Microservices with Node.js: Key Insights
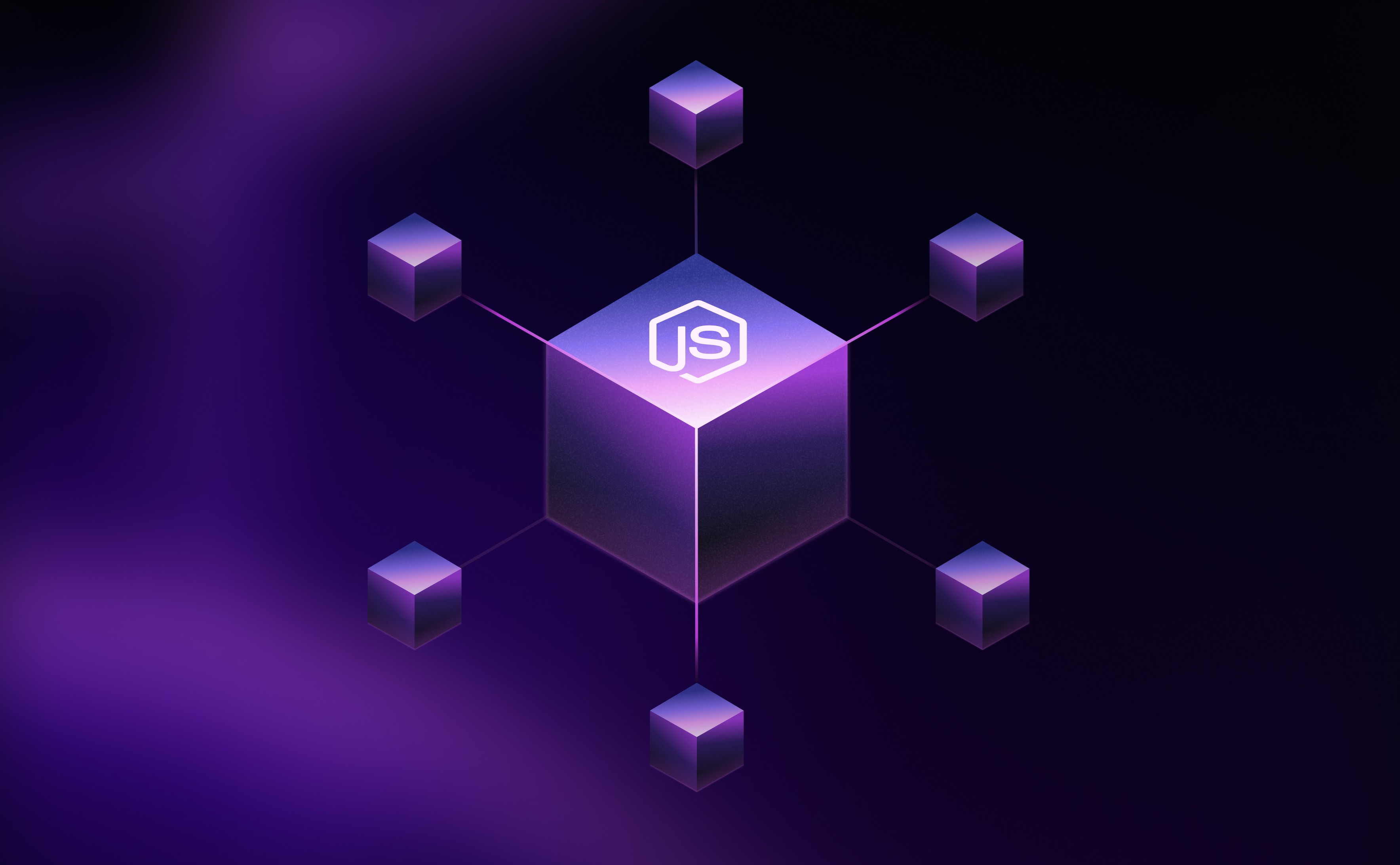
When Uber began experiencing explosive growth, they quickly realised their monolithic architecture was struggling to keep pace. According to Uber’s blog on service-oriented architecture, the company encountered several critical issues before transitioning to microservices, such as:
- Difficulty scaling rapidly: Accommodating huge spikes in rider demand worldwide was cumbersome within a monolithic setup.
- Slow deployment cycles: Rolling out new features or fixes often meant extensive code changes and lengthy testing procedures.
- Complex codebase management: Many teams working on a single codebase led to frequent merge conflicts.
To overcome these issues, Uber adopted a microservices architecture, breaking down their core system into smaller, independently deployable services.
To overcome these issues, Uber adopted a microservices architecture, breaking down their core system into smaller, independently deployable services.
The success of this shift at Uber isn’t an isolated story—companies worldwide, including Amazon, Netflix, SoundCloud, and Twitter, are adopting microservices to break free from the constraints of monolithic systems.
A 2020 O’Reilly survey on microservices adoption polled over 1,500 technology professionals—including executives, engineering managers, and software architects—revealing that the majority were already using or planning to adopt microservices, highlighting its growing adoption across both leadership and technical roles.
Their top motivations included:
- Accelerating feature development
- Simplifying scalability
- Enhancing organisational agility
Whether you’re looking to streamline deployment, improve fault isolation, or simply make your team more agile, this guide will walk you through the essentials of developing microservices with Node.js.
Understanding microservices architecture
Microservices architecture organises a software system into multiple independent services, each responsible for a specific task. Rather than running everything under one large application, it breaks tasks into smaller units that can be developed, tested, and deployed on their own schedule. This contrasts with the traditional “monolithic” approach, where the entire application is bundled into a single, large codebase.
Key characteristics of microservices
1. Independent services: Each microservice has its own codebase, data storage, and deployment pipeline. This separation limits the impact of errors to a single service, preventing issues from cascading across the entire system.
2. Decentralised data: Microservices can use different databases or storage technologies tailored to the unique needs of each service. This flexibility can improve performance, scalability, and overall business agility.
3. Lightweight communication: Services typically communicate via REST APIs, message queues, or gRPC. This approach minimises overhead, supports seamless integration, and allows each service to evolve independently.
4. Targeted scalability: Because each service is autonomous, you can scale the components facing the highest demand without over-allocating resources to other parts of the system. This can reduce costs and speed up response times.
5. Dedicated teams: Businesses often assign a small, cross-functional team to each microservice. This clear ownership streamlines development cycles, keeps accountability high, and improves responsiveness to customer needs.
Microservices enable faster innovation and a more resilient technology stack. By reducing bottlenecks and isolating risks, they create an environment where new features can be rolled out on demand and critical services remain stable under heavy load.
At a glance, microservices architecture also proves beneficial for anyone seeking to modernise large systems, breaking them down into manageable parts that can evolve smoothly over time.
Wondering if microservices architecture is right for you?
Let’s talkLoading...
Monolithic vs Microservices in Node.js
When building with Node.js, you can choose to bundle your entire application into a single codebase (monolithic) or split it into smaller, independent services (microservices). Although Node.js performs well in both setups, each approach has its own advantages and hindrances.
Aspect | Monolithic architecture | Microservices architecture |
---|---|---|
Codebase | Single repository containing all features and logic | Multiple repositories, each focused on a specific service |
Deployment | Single deployment pipeline, simpler to set up | Separate pipelines for each service, allowing independent updates |
Scalability | Scale by replicating the entire application | Scale individual services based on demand, optimising resource usage |
Fault Isolation | Issues can affect the entire application | Failures are isolated to one service, reducing the impact on the overall system |
Team Coordination | Requires careful coordination among all contributors in one codebase | Smaller, focused teams can develop and deploy services independently |
Updates & Releases | Rolling out new features or bug fixes may involve the entire codebase | Changes can be deployed to a single service without affecting others |
Complexity | Easier to start with but can become unwieldy as the application grows | Requires solid planning to manage inter-service communication, but remains more flexible over time |
Performance | Node.js remains fast, but a monolith might be harder to optimise at scale | Distributing loads among services helps maintain performance under varying traffic patterns |
The table below outlines the primary differences to help you choose the right architecture for your project.
When choosing between monolithic and microservices, consider the size of your project, the structure of your development teams, and your long-term scalability goals. Smaller or more focused applications may thrive under the simplicity of a monolith, while larger or rapidly evolving projects often benefit from microservices’ flexibility and isolation.
Why choose Node.js for your microservices architecture?
Node.js stands out for building microservices because of its lightweight, event-driven nature and vast ecosystem of tools.
Here are the key reasons to consider it:
- Asynchronous, non-blocking I/O: Node.js handles multiple requests without tying up resources. This helps each microservice remain responsive under a heavy load.
- Vast NPM ecosystem: With access to over a million packages, teams can rapidly integrate and customise various functionalities—reducing development time for each service.
- Unified language: Using JavaScript on both front-end and back-end fosters shared knowledge across teams. This streamlined communication can speed up feature development and reduce onboarding time.
- Lightweight deployment: Node.js apps have a small memory footprint, allowing microservices to run efficiently on different machines or containers.
- Strong community support: The active Node.js community offers extensive documentation, libraries, and best-practice guides to help maintain and evolve a microservices setup over time.
By leveraging these benefits, teams can build microservices that perform well, scale with demand, and stay flexible as business needs evolve.
Common challenges in Node.js microservices
Microservices can introduce operational and development complexities, even when using a lightweight runtime like Node.js.
Below are the most common challenges teams face and practical ways to tackle them.
1. Communication overhead
Coordinating messages between multiple Node.js services can quickly become complex. Although Node.js’s asynchronous I/O model handles concurrent requests efficiently, connecting many services requires careful API design, load balancing, and error-handling strategies.
How to overcome:
- Clear API contracts: Define well-documented REST or RPC endpoints so each service knows exactly what to expect.
- Message queues or event-driven patterns: Tools like RabbitMQ or Kafka can buffer and distribute messages, reducing direct dependencies between services.
- Circuit breakers & retries: Implement patterns that prevent individual service failures from cascading throughout the system.
2. Debugging distributed systems
Finding the source of an error in a single monolithic application can be straightforward, but tracing it through a network of Node.js microservices is more involved. Logs from multiple services and machines must be correlated to see the bigger picture.
How to overcome:
- Centralised logging: Aggregate logs in one place using platforms like ELK (Elasticsearch, Logstash, Kibana) or cloud-based logging services.
- Distributed tracing tools: Solutions such as Jaeger or Zipkin let you follow requests across service boundaries, pinpointing failures more quickly.
3. Maintaining data consistency
Each microservice typically owns its own data store, which simplifies local operations but complicates transactions that span multiple services. Consistent state across these services becomes harder to guarantee.
How to overcome:
- Event-driven architecture: Use events to notify other services of changes. Each service then updates its own data as needed.
- Compensating transactions: Implement fallback actions to revert partial operations if one part of a multi-service transaction fails.
- Idempotent operations: Ensure that repeating a transaction or event multiple times won’t create inconsistent data.
4. Monitoring and scaling
Although Node.js applications are resource-friendly, running numerous services means managing CPU usage, memory consumption, and performance metrics across different containers or servers. Undetected bottlenecks can degrade overall performance.
How to overcome:
- Comprehensive monitoring stack: Employ tools like Prometheus, Grafana, or Datadog to collect and visualise metrics across services.
- Autoscaling policies: Configure cloud or container orchestration platforms (e.g., Kubernetes) to spin up or scale down services based on real-time demand.
- Alerting and incident response: Set thresholds for key metrics and alert on anomalies before they impact users.
By recognising these challenges early and implementing robust solutions, teams can design Node.js microservices that harness concurrency benefits, scale effectively, and remain resilient under heavy load.
Popular microservices Node.js frameworks
Several Node.js frameworks can help streamline the process of developing and managing microservices. Some offer a comprehensive toolset for service discovery, inter-service communication, and fault tolerance, while others provide a lighter foundation you can build upon.
Here are a few popular choices:
NestJS
- TypeScript-centric: Built with TypeScript, NestJS enforces strong typing and modular structure by default.
- Opinionated architecture: Encourages the use of controllers, services, and modules, making it easy to break your application into microservices.
- Built-in microservices support: Offers transport layers for TCP, Redis, gRPC, and more. This allows you to configure service-to-service communication without extra libraries.
Moleculer
- Designed for microservices: Focuses on features like service discovery, load balancing, event-driven architecture, and fault tolerance.
- Out-of-the-box tools: Provides a built-in logger, transporter modules (NATS, Redis, Kafka), and other components to reduce boilerplate.
- Pluggable approach: This lets you integrate various custom or third-party modules to extend your microservices’ functionality.
Seneca
- Plugin architecture: Encourages building functionality through small, reusable plugins.
- Pattern-based: Services interact by calling actions defined by patterns, simplifying request/response logic.
- Minimal boilerplate: Ideal if you prefer a lightweight framework that you can extend as your application grows.
Express
- Familiar foundations: Although not a dedicated microservices framework, Express remains the most widely used Node.js web framework.
- Microservice patterns: You can split functionalities into separate services and add libraries for service discovery, messaging, and monitoring.
- Flexibility: Ideal for teams already comfortable with Express who want to gradually migrate to a microservices architecture without a steep learning curve.
Which one to choose?
It depends on your team’s familiarity with each framework, your need for out-of-the-box microservices features, and how structured or “opinionated” you want your architecture to be.
If you want a dedicated system for microservices, frameworks like Moleculer, Seneca, or NestJS can reduce the time spent on building common features.
If you’re more comfortable with a simpler approach, Express offers the flexibility to integrate microservice patterns at your own pace.
Best practices for developing Node.js microservices
Building microservices architecture in Node.js isn’t just about splitting code into smaller services. It requires strategic planning and consistent implementation of proven patterns.
Below are key best practices to keep your node microservices efficient, reliable, and easy to maintain:
1. Keep microservices loosely coupled
Each microservice should handle a single domain or function, minimising dependencies on other services. This isolation ensures that failures remain contained and updates can be rolled out independently.
2. Follow a consistent code structure
Standardise folder layouts, naming conventions, and project setup across all services. This uniformity makes it easier for developers to move between microservices and maintain code quality.
3. Use environment variables and configuration management
Store environment-specific settings—like database URLs or API keys—in .env files or a secret manager. Avoid hardcoding credentials or environment details to keep deployments portable and secure.
4. Implement security and authorisation
Use JWT or another secure token-based mechanism to protect sensitive endpoints. Validate requests thoroughly, especially when services expose public-facing APIs.
5. Centralise logging and monitoring
Centralised logs and metrics offer an overview of your system’s health. Tools like ELK (Elasticsearch, Logstash, Kibana) or cloud-based platforms help you trace issues across multiple Node.js services in one place.
6. Embrace observability
Distributed tracing solutions like Jaeger or Zipkin let you follow the lifecycle of requests that hop between services. Early integration of tracing and metrics simplifies debugging as your system grows.
7. Automate testing and CI/CD
Implement unit tests, integration tests, and end-to-end tests for each microservice. Coupled with continuous integration/continuous deployment (CI/CD), these tests help catch issues early and maintain a reliable release process.
8. Use resilience patterns
Protect your services from cascading failures with circuit breakers, timeouts, and retries. These patterns prevent one malfunctioning service from degrading others.
9. Manage service discovery and routing
Use a service registry or an API gateway to handle communication among microservices. This approach abstracts away service locations, making it easier to add, remove, or scale individual services.
10. Document APIs and version endpoints
Clear documentation (e.g., OpenAPI/Swagger) and versioning help teams know exactly how to consume each service’s endpoints. Versioning also prevents breaking changes from affecting existing integrations.
By adhering to these best practices, you’ll foster an environment where Node.js microservices can evolve rapidly without sacrificing reliability or performance.
Need a custom Node.js microservices solution?
Reach out to us!Loading...
Bottom line
Microservices using Node.js empower you to break large, monolithic applications into smaller, independently deployable components. By structuring your services around specific business functions and leveraging Node.js’s performance strengths, you can build systems that scale smoothly and adapt quickly to new requirements.
While microservices add complexity—especially in areas like communication, data consistency, and monitoring—they offer benefits like targeted scalability and improved fault isolation.
By applying the best practices and using established frameworks, you can develop resilient, high-performing Node.js microservices that serve your users and your organisation’s goals effectively.
Webandcrafts (WAC) is a Node.js development company that provides high-quality software development solutions, specialising in building innovative and scalable applications for businesses across industries. With our expertise in Node.js development services, WAC helps your organisation implement efficient, high-performance microservices architectures that scale seamlessly and drive business growth. Hire a Node.js developer at WAC to build scalable and resilient systems.
Looking to modernize your application with Node.js?
Contact us today!Loading...
Discover Digital Transformation
Please feel free to share your thoughts and we can discuss it over a cup of tea.