Guide to Android App Architecture
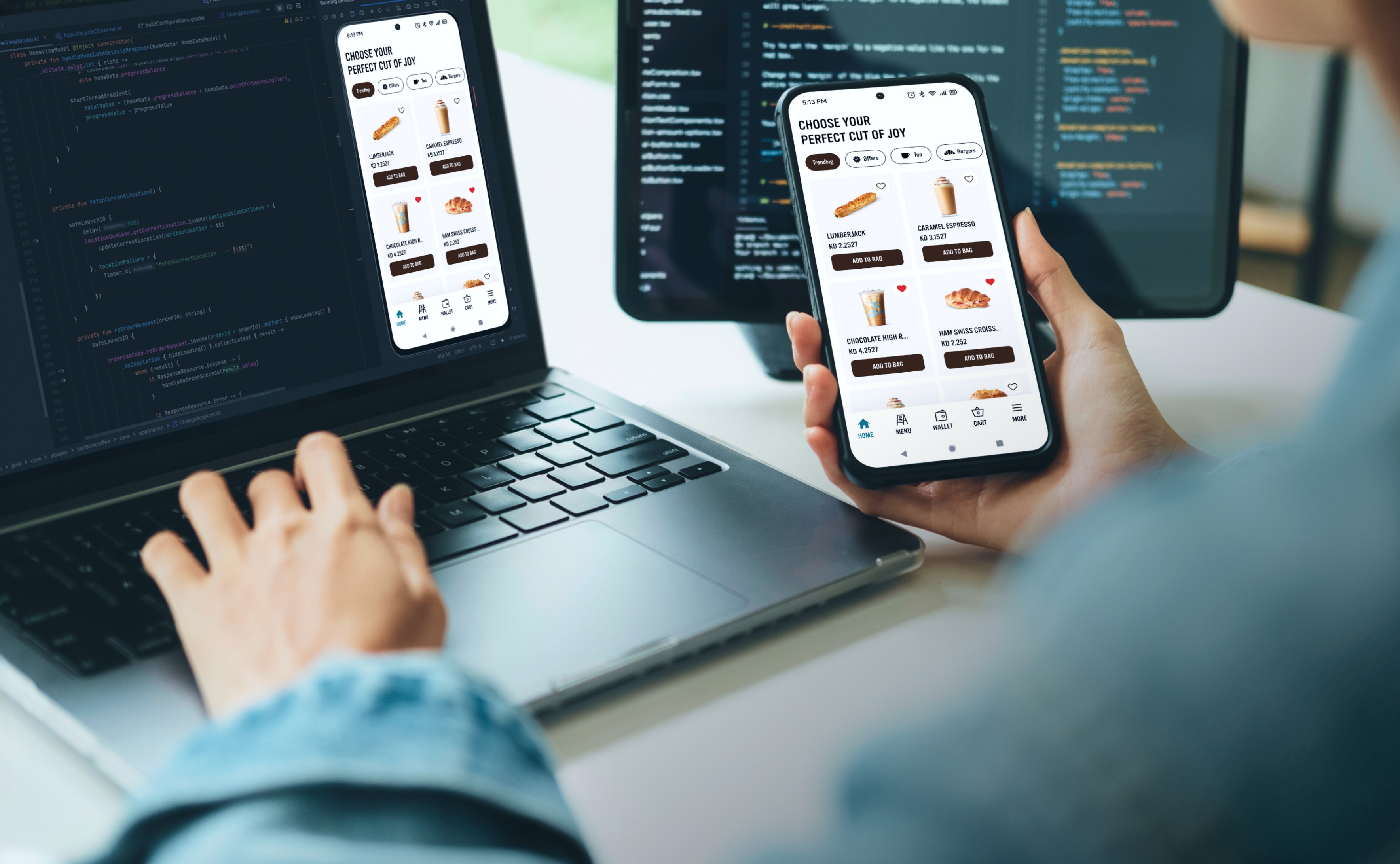
The way an Android application is structured plays a crucial role in how well it performs, how easily it can grow, and how maintainable it is over time. Investing in a robust Android app architecture is crucial for delivering a smooth user experience and achieving lasting success. As mobile applications grow more intricate, having a clear development process can significantly boost business growth.
New Android architecture components simplify the development process, improve app performance, and provide security. Studies indicate that the majority of mobile users abandon an application due to slow performance, a clear indication that there is a need for a robust Android platform architecture.
This article covers Android application architecture, its main layers, most commonly used architectural patterns, and the strategic benefits of well-designed architecture for big applications.
What is Android App Architecture?
The architecture of Android is similar to a carefully thought-out blueprint that specifies how various components interact. Its goal is to create applications that are not only user-friendly but also easy to expand, ensuring that data flows seamlessly throughout the system.
Android application architecture is composed of various layers that differentiate concerns and thus develop, test, and maintain sophisticated applications efficiently. An Android app architecture with clear definitions allows developers to design stable applications that meet business objectives.
Android App Experience
Android apps run in a dynamic environment where system resources vary with utilization. Android platform architecture comprises critical elements such as:
- Activities (User interface screens)
- Fragments (Reusable UI components)
- Services (Background tasks)
- Broadcast Receivers (System-wide event handlers)
- Content Providers (Data sharing mechanisms)
As Android maximizes system performance by closing down idle processes, a robust Android app design is necessary to ensure seamless user flows at all times. The app states that being preserved regardless of system limitations is of utmost concern to business owners, as it is directly related to user retention and satisfaction.
Layers of Modern Android Application Architecture
A well-structured Android application architecture consists of three primary layers:
1. UI Layer
The UI layer is essentially the appearance of the app. The UI layer of the app is what renders information on the screen and responds to actions like taps, swipes, and gestures. Not only should a good UI feel right and look nice, but it also needs to make the app more intuitive, responsive, and easy to use.
The following are the most critical elements of this layer:
- Activity/Fragment
Activities and Fragments are the fundamental components of Android UI development. An Activity is a user interface screen, and it is a portal for user interaction. It manages various lifecycle events such as creation, pause, resume, and destruction. Yet, a Fragment is a container for part of an Activity's user interface, so that more reusable and dynamic UI elements that can fit into different screen sizes and orientations can be achieved.
- ViewModel
The ViewModel is what isolates UI logic from the actual UI controls, so the design is clean and maintainable. This technique makes it easy to manage configuration changes, like when you rotate your screen, without having to reload data unnecessarily. It keeps the UI-related data in sync with the app's lifecycle. In essence, the ViewModel is an interface or bridge between the data layer and UI elements like Activities and Fragments, ensuring that the UI updates are quick and seamless.
- State Management
State management holds UI state data, e.g., in the event of loading, success, and error status, preventing flicker or unpredictable update of UI elements by providing a structured management of state change. Good state management can enhance performance by only updating the necessary UI components instead of redrawing the whole screen.
2. Domain Layer
The Domain Layer is the heart of an Android app's architecture. You can think of it as the crucial link that manages all the business logic connecting the data layers to the user interface. By keeping the application logic separate from external dependencies, it becomes a lot simpler to scale, test, and maintain the software. To ensure the application delivers a seamless and consistent user experience, this layer needs to effectively process, modify, and implement the data.
There are three key components to consider:
- Use Cases
Use cases outline the specific procedures or actions that an application carries out, making them invaluable for designing business logic.
Every Use Case corresponds to one operation, i.e., getting user data, making payments, or giving discounts. By encapsulating logic within defined Use Cases, developers write code that is modular, reusable, and less prone to breaking when the app is updated, thereby making it easier to maintain as the application ages.
- Entities
Entities are the fundamental business models that capture real-world things and ideas in the application. They are basic, data-containing objects that specify the form of important business-related data, e.g., User, Product, Order, or Transaction. Contrary to database models, Entities are confined within the Domain Layer as clean business representations and are not dependent on data storage or retrieval mechanism changes. Thanks to this approach, business rules remain protected from any changes that might come from shifts in data sources or storage methods.
- Repositories
Repositories serve as a crucial link between the Data Layer and the Domain Layer, overseeing how data flows from different sources like local databases, remote APIs, or even in-memory caches. They simplify access to data for Use Cases, allowing developers to focus on what they need without getting bogged down by the nitty-gritty of how the data is implemented. This level of abstraction offers great flexibility, enabling developers to swap out or modify data sources without disrupting the essential business logic.
When you set up the Domain Layer the right way, Android apps turn into something much easier to manage, grow, and adapt as needs change. This clear division of responsibilities results in cleaner code, improved performance, and a smoother development journey.
3. Data Layer
This layer is accountable for dealing with data of an Android application, whether it is from a remote server, a local database, or even cache memory. This layer only cares about making sure data is fetched, stored, and delivered to the Domain Layer for processing of business logic in the most optimal way possible. By separating data management from the rest of an application, developers can build apps that are not only scalable but also easier to test and maintain.
This layer includes three components:
- Network Data Source
The Network Data Source is accountable for retrieving data from external web services, cloud storage, or APIs. To get information quickly, it handles API calls and network searches. For these kinds of tasks, developers often use libraries like OkHttp, Retrofit, or Volley. But if the network or server is slow, the request might not go through. This means that error handling is needed to make sure the customer doesn't get a bad experience.
- Local Database
The Local Database provides a reliable way to store application data, ensuring that users can access their information even when they're offline. It typically uses Room (which is an abstraction over SQLite), SharedPreferences, or ObjectBox to keep structured data safe. The Local Database enhances responsiveness and provides a seamless experience by keeping frequently used data close at hand, which helps reduce those pesky unnecessary API requests.
- Repository
The Repository serves as a bridge between the Network Data Source and the Local Database, managing the way data is accessed and where it comes from. It encloses the sources of the data so that business logic in the Domain Layer has no idea if the data comes from a database, a local cache, or a remote server. This separates the parts of the application, making it easier to switch or change data sources without affecting other parts of the program.
Components of Android Architecture
The Android platform's multi-layered architecture offers robust security, performance, and a seamless user experience. It is made up of several major parts:
Applications
These are the user applications that leverage Android's underlying services and features. Each application runs in its own process, which aids in security and stability.
Application Framework
This layer provides essential APIs and services that developers rely on to create Android applications. It includes UI components, content providers, activity management, resource management, and system services—valuable tools that make application development much easier.
Android Runtime (ART)
The Android Runtime (ART) runs app code and handles memory. To increase performance, trash collection, and execution speed, it replaces the outdated Dalvik Virtual Machine (DVM) and uses Ahead-of-Time (AOT) compilation rather than Just-in-Time (JIT) compilation.
Platform Libraries
Android provides a number of pre-built libraries to facilitate media processing, graphics rendering, security, database administration, and networking. These libraries save developers from having to start from scratch by allowing programs to handle complex tasks.
Linux Kernel
The core of Android architecture relies on the Linux Kernel. It manages essential system functions such as process management, memory management, hardware abstraction, security, and power management. The Linux Kernel provides stability, security, and smooth communication between the hardware and the software layers.
With the knowledge and proper organization of these layers, developers can design efficient, scalable, and high-performance Android apps that deliver a smooth user experience while maintaining long-term maintainability.
Time to Upgrade Your App’s Architecture?
Reach out to usLoading...
Popular Android App Architecture Patterns
There are several architectural patterns employed in the development of Android applications:
1. MVC (Model-View-Controller)
The MVC (Model-View-Controller) pattern is one of the oldest and easiest architectural patterns employed in software development. In this:
The Model is the data layer and business logic.
- The View is tasked with controlling the user interface controls and how the users interact with them.
- Playing the role of the mediator, the Controller translates the user input and makes sure the Model and the View are changed accordingly.
Pros:
- Rapid setup – Ideal for those projects that require quick development but not much complexity.
Cons:
- Tight coupling of the parts – View and Controller get coupled, making it difficult to decouple concerns.
- Maintenance difficulty – With the project growing, interdependencies between Controller and View complicate updating and testing the codebase.
Best used for: Tiny applications with little UI logic and simple interactions.
2. MVP (Model-View-Presenter)
The MVP (Model-View-Presenter) pattern continues the MVC concept by introducing a Presenter that serves as an intermediary between the Model and the View. This setup helps maintain the user interface logic and the business logic separate from each other, thus making it a cleaner and more organized design.
- The Model does all the data-related operations.
- The View is responsible for presenting the user interface and communicating with the user.
- The Presenter takes care of the business logic and updates the View whenever needed.
Pros:
- Improved separation of concerns – Since the Presenter manages the UI logic, the View is simpler and far less complicated to manage.
- Easier testing – Unit testing is easier through the isolation of business logic from UI elements.
Cons:
- More complex than MVC – This method is slightly more complicated than MVC because it adds an additional Presenter layer, which takes more work to implement and maintain.
Best for: Mid-sized applications that strike a balance between being easy to maintain, especially those that involve some UI logic.
3. MVVM (Model-View-ViewModel)
MVVM (Model-View-View-Model) is a newer pattern for Android application architecture, optimized for state management and UI separation.
- The Model holds business logic and data sources.
- The ViewModel takes care of data and business logic associated with the UI, so the View simply focuses on presenting information.
- The View waits for changes to the ViewModel and then updates the UI.
Pros:
- Enables UI state management – ViewModel stores data even in the presence of configuration changes such as screen rotation.
- Enhances testability – Because the ViewModel is independent of the UI, unit testing is improved.
Cons:
- Steep learning curve – The steepness of the learning curve comes in because the developers have to learn ViewModel, LiveData, and data binding, making it more complicated.
Best for: Scaling and maintaining programs, particularly those involving real-time data synchronization and dynamic user interface rendering.
4. MVI (Model-View-Intent)
By applying a one-way data flow, MVI (Model-View-Intent) streamlines state management to be more scalable and more reliable.
- The Model tightly couples application state and business logic.
- The View monitors for any change and redraws the user interface as a result.
- The View captures user interactions as Intents, which are processed to update the Model. Changes in the Model are then reflected back in the View.
Pros:
- One-way data flow simplifies debugging and helps prevent unexpected UI states by ensuring that data changes move in a single, predictable direction.
- Centralized state improves consistency and readability, streamlining overall state management.
Cons:
- Expertise required – To apply MVI successfully, you actually need to have good knowledge of RxJava, Kotlin Coroutines, and event-driven architecture.
Best for: Large applications with complex user interfaces, such as those with dynamic animation, real-time updates, or many users interacting simultaneously.
Considerations for Large-Scale Android Apps
Clean Architecture
A clean architecture is essential for long-term scalability, maintainability, and efficiency in large commercial Android apps. By adhering closely to SOLID principles, Clean Architecture highlights the significance of modularity, testability, and maintainability. It skillfully separates various concerns, minimizes dependencies, and allows for the seamless addition of new features without interfering with existing functionality.
Layers
Large apps have three main layers:
- Data Layer – All data-related activities, including information retrieval and storage, happen here.
- Domain Layer – This layer concerns the business logic and the critical functions of the app.
- UI Layer – This section handles everything that users will view and use.
This structure makes it easier to find bugs, keep the code up to date, and make changes that don't affect the whole app.
Modules
Breaking the app into smaller modules keeps things clean. While base modules manage general tasks required throughout the app, feature modules are devoted to individual features. Moreover, library modules include reusable tools or components. This setup speeds up development and makes it easier for teams to collaborate.
Scalability & Performance
To keep the app running smoothly even under high traffic, memory use, database access, and background tasks are optimized. Tools like dependency injection help manage resources more effectively.
Testing & Integration
Automated tests, such as unit tests and UI tests, make sure the app functions as expected. Continuous Integration/Continuous Deployment (CI/CD) workflows detect problems early and maintain software stability.
With these, businesses can create scalable, high-quality Android apps that are sustainable, flexible, and future-proof.
Bottom Line
A well-designed Android app architecture is the foundation of any successful mobile app. Not only does it increase performance and scalability, but it also improves maintenance and user satisfaction. By clearly separating concerns across the UI, Domain, and Data layers, developers can ensure smooth data flow, modular code, and flexibility in adapting to future requirements. By including architecture components such as ViewModel, Repositories, and Use Cases, they can enable improved state handling and keep business logic safe from implementation changes.
Though every architectural pattern—MVC, MVP, or MVVM—has its pros and cons, choosing the right one depends on the size and complexity of the project. But the key is to pick the architecture as per the long-term objective and technical requirements of the app.
As a leading Android development company, WAC understands that the key to any successful app begins with proper architecture. Our developers create Android apps that are not only scalable and dependable but also optimized to provide users with a seamless, interactive experience. Looking to strengthen your mobile team? You can hire dedicated Android developers from WAC for a specific task or build a full team to accelerate your app development. Contact us today!
Ready to Scale Your Android App?
Let's talkLoading...
Discover Digital Transformation
Please feel free to share your thoughts and we can discuss it over a cup of tea.